showattitude
¶
Source code:: showattitude
SHOW Attitude Solution¶
This module is used to generate the attitude solution required for the SHOW data from the attitude data measured by the ER2 aircraft. The attitude solution is typically injected into the processing stream between Level 1A and Level 1B.
Analysis of the SHOW instrument data requires the position and orientation in space of the ER2 aircraft to be known for each exposure taken by SHOW during the ER2 flight. Once the aircraft orientation in space is known an additional rotation matrix provides the instrument lines of sight. The ER2 attitude solution is provided by the ER2 NASDAT system which provides a series of one-line, text records each providing an array of csv (comma separated values):
IWG1Collection,2017-04-22T20:22:19.004,33.897535392,-87.538252378,19341.8,19341.8,62947.5,,197.3,200.3,,0.689,386.0,-45.80,315.5,1.3,1.76,-0.35,,,-62.4,,-42.4,62.3,23.2,315.7,5.5,256.1,,40.7,48.6,-112.4,-64.4 IWG1Collection,2017-04-22T20:22:20.004,33.898800291,-87.539853058,19343.9,19343.9,62955.0,,197.2,200.1,,0.689,416.0,-45.81,315.5,1.4,1.80,-0.26,,,-62.7,,-42.7,62.2,23.2,315.2,5.5,255.9,,40.7,48.5,-112.4,-64.4 IWG1Collection,2017-04-22T20:22:21.004,33.900064725,-87.541454323,19346.1,19346.1,62970.0,,197.1,199.9,,0.688,416.0,-45.83,315.5,1.4,1.72,-0.29,,,-62.7,,-42.7,62.2,23.1,314.7,5.5,253.6,,40.7,48.6,-112.4,-64.4This format is known as IWG1Collection format and is described on the Nasa Dryden ER2 website. The fields of interest to us, along with column index and value extracted from first line of example above are,
- Timestamp (1), 2017-04-22T20:22:19.004
- Latitude (2), 33.897535392
- Longitude (3), -87.538252378
- GPS_Alt_MSL (4), 19341.8
- True_Heading (13), -45.80
- Pitch_Angle (16), 1.76
- Roll_Angle (17), -0.35
(i) Global Geocentric Coordinates¶
The global coordinate system with the origin at the centre of the Earth. This is a right-handed, 3 axis system with
- \(\hat{x_g}\) in the equatorial plane pointing to \(0^{\circ}\) longitude.
- \(\hat{y_g}\) in the equatorial plane pointing to \(90^{\circ}\) longitude.
- \(\hat{z_g}\) parallel to the Earth’s spin axis.
The Earth is assumed to be an oblate spheroid given by the WGS84 specification. This geoid has been intentionally chosen to match the GPS system used to generate the ER2 latitude, longitude and altitude coordinates.
(ii) Topocentric Coordinates¶
This is 3 axis, right-handed, orthogonal coordinate system defined for each latitude and longitude on the oblate spheroid. The local topocentric coordinates are:
- \(\hat{\texttt{north}}\)
- \(\hat{\texttt{east}}\)
- \(\hat{\texttt{down}}\)
The 3 unit vectors are readily expressed in terms of the global geocentric unit vectors given the latitude and longitude of the ER-2 aircraft measured by the GPS system. Note that we have chosen unit vectors that match the definition normally used in aviation but is opposite to the topocentric unit vectors produced by the sasktran code.
(iii) Aircraft Reference Frame¶
The aircraft is arbitrarily oriented in the topocentric system and is fully specified by the orientation of 3 unit vectors:
- \(\hat{\texttt{nose}}\), parallel to the aircraft body pointing forwards through the nose.
- \(\hat{\texttt{starboard}}\), parallel to the starboard wing.
- \(\hat{\texttt{wheels}}\) parallel to the wheels in the downward direction.
(iv) True Heading, Pitch and Roll¶
The aircraft orientation in space is specified by the IWG1Collection fields, True Heading, Pitch and Roll which are applied as three rotations. Start the rotations with \(\hat{\texttt{nose}}\), \(\hat{\texttt{starboard}}\) and \(\hat{\texttt{wheels}}\) aligned with \(\hat{\texttt{north}}\), \(\hat{\texttt{east}}\) and \(\hat{\texttt{down}}\) respectively.
- Apply a right-handed rotation around the current \(\hat{\texttt{wheels}}\) axis of True Heading degrees.
- Apply a right-handed rotation around the current \(\hat{\texttt{starboard}}\) vector of Pitch degrees.
- Apply a right handed rotation around the current \(\hat{\texttt{nose}}\) of Roll degrees.
The aircraft orientation in space is now described by the current orientation of \(\hat{\texttt{nose}}\), \(\hat{\texttt{starboard}}\) and \(\hat{\texttt{wheels}}\) unit vectors.
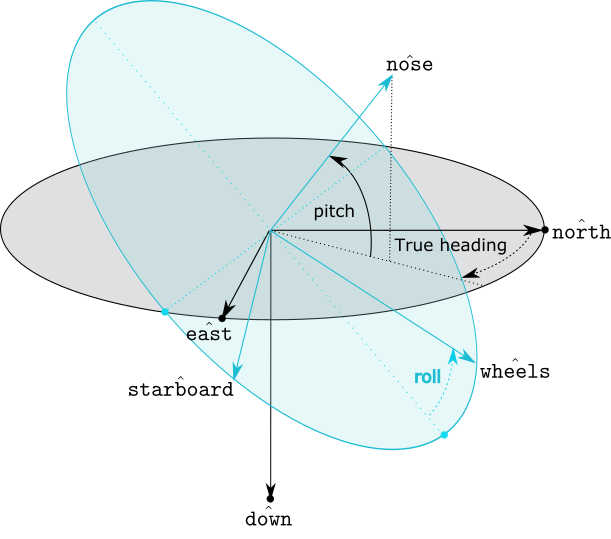
Class IWG1¶
-
class
showattitude.iwg1.
IWG1
(utc: numpy.datetime64 = nan, latitude: float = nan, longitude: float = nan, altitude: float = nan, pitch: float = nan, roll: float = nan, heading: float = nan)[source]¶ Instance Attributes:
-
utc
¶ np.datetime64 representing the UTC time of the attitude record.
-
latitude
¶ The geodetic latitude of the aircraft in degrees North
-
longitude
¶ The geodetic longitude of the aircraft in degrees East.
-
altitude
¶ The altitude of the aircraft above mean sea level in meters.
-
pitch
¶ The pitch of the aircraft in degrees.
-
roll
¶ The roll of the aircraft in degrees.
-
heading
¶ The true heading of the aircraft in degrees. 0 degrees in North, 90 degrees is East, 180 is South and 270 is West.
-
location
¶ A 3 element array. The geocentric vector location of the aircraft in meters from the center of the earth. The coordinates of the vector are, x= 0, y = 1 and z = 2.
-
north
¶ A 3 element array. The geocentric unit vector representing geographic north at the aircraft location. The coordinates of the unit vector are x= 0, y = 1 and z = 2.
-
east
¶ A 3 element array. The geocentric unit vector representing geographic east at the aircraft location. The coordinates of the unit vector are x= 0, y = 1 and z = 2.
-
down
¶ A 3 element array. The geocentric unit vector representing geographic down at the aircraft location. Do not confuse this field with the
wheels
attribute which is typically close to down when the plane is flying horizontally. The down unit vector is independent of the aircraft and only depends upon the shape of the Earth. The coordinates of the down unit vector are x= 0, y = 1 and z = 2.
-
nose
¶ A 3 element array. The unit vector of the x axis of the aircraft reference frame expressed in geocentric coordinates. This is known as the aircraft nose direction. The coordinates of the unit vector are x= 0, y = 1 and z = 2.
-
starboard
¶ A 3 element array. The unit vector of the y axis of the aircraft reference frame expressed in geocentric coordinates. This is known as the aircraft starboard direction. The coordinates of the unit vector are x= 0, y = 1 and z = 2.
-
wheels
¶ A 3 element array. The unit vector of the z axis of the aircraft reference frame expressed in geocentric coordinates. This is known as the aircraft wheels direction. The coordinates of the unit vector are x= 0, y = 1 and z = 2.
-
Class IWG1Collection¶
This is a class that stores a collection of IWG1 lines. The current implementation focusses only on the fields required for the SHOW attitude solution and makes no attempt to support the multitude of other variables broadcast in each IWG1Collection record.
Methods¶
The full list of methods is given below but you might find the following links to the most important methods to be convenient
concatentate()
at()
interpolate()
-
class
showattitude.iwg1.
IWG1Collection
(filename: str = None, listoflines: typing.List[str] = None, url: str = None)[source]¶ Decodes the IWG1Collection records generated by the NASA ER-2 system. The current code only extracts information required to determine aircraft location and orientation. Records with invalid fields are rejected during the decode process. Several options are available during construction to initialize the records but by default the arrays will be empty. Use of the keyword arguments allows the constructor to initialize the internal arrays.
Instance Attributes:
-
utc
¶ A 1-D array(npts) of datetime representing the UTC time of each attitude record.
-
latitude
¶ A 1-D array(npts). The geodetic latitude of the aircraft in degrees North
-
longitude
¶ A 1-D array(npts). The geodetic longitude of the aircraft in degrees East.
-
altitude
¶ A 1-D array(npts). The altitude of the aircraft above mean sea level in meters.
-
pitch
¶ A 1-D array(npts). The pitch of the aircraft in degrees.
-
roll
¶ A 1-D array(npts). The roll of the aircraft in degrees.
-
heading
¶ A 1-D array(npts). The true heading of the aircraft in degrees. 0 degrees in North, 90 degrees is East, 180 is South and 270 is West.
-
concatenate
(other: showattitude.iwg1.IWG1Collection)[source]¶ Concatenates the given array of records onto the current array. No attempt is made to ensure the concatenated records are in sequential order”.
Parameters: other – Another instance of WIG1 that will concatenated to this instance.
-
from_file
(filename: str)[source]¶ Creates an array of records from IWG1Collection records read in from a file. Any previous records are erased.
-
from_lines
(listoflines: typing.List[str])[source]¶ Creates an array of records from a list of IWG1Collection text records. Any previous records are erased.
-
from_url
(url: str)[source]¶ Creates an array of records from IWG1Collection records read in from a URL. Any previous records are erased. Note that no timeouts are applied to the URL read so this call meust be used with caution in time-critical systems.
-
interpolate
(utcnow: typing.Union[datetime.datetime, numpy.datetime64], extrapolate: bool = False, truncate: bool = False, maxgap_seconds=None)[source]¶ Linearly interpolates the IWG1Collection tables and returns the IWG1 corresponding to the interplated time.
Parameters: - utcnow – The time at which the attitude solution is required
- extrapolate – Optional parameter. If True then linearly extrapolate beyond the start and end of the collection
- truncate –
- maxgap_seconds –
Returns:
-
Class WGS84¶
-
class
showattitude.wgs84.
WGS84
[source]¶ Implements a few geodetic conversion functions using the WGS 84 ellipsoid. This is identical to ISKGeodetic WGS84 option in the sasktran libraries but is coded up in pure pytho
-
classmethod
geodetic_to_geocentric
(latitude: typing.Union[float, numpy.ndarray, typing.List[float]], longitude: typing.Union[float, numpy.ndarray, typing.List[float]], altitude: typing.Union[float, numpy.ndarray, typing.List[float]]) → typing.Tuple[[numpy.ndarray, numpy.ndarray, numpy.ndarray], numpy.ndarray][source]¶ This function conevrts geodetic latitude, longitude and altitude into geocentric x y and z coordinates using the WGS84 ellipsoid. It also returns the unit vectors for north, east and down at the location. The implementation will accept scalar or 1-D array based valuesr
Parameters: - latitude – The geodetic latitude in degrees. Either scalar or list/array.
- longitude – The geodetic longitude in degrees. Either scalar or list/array, must be same size as latitude
- altitude – The altitude above mean sea level in meters. Either scalar or list/array, must be same size as latitude.
Returns: A tuple of 4 arrays ( location, north, east, down)
- location is the geocentric, xyz, vector location in meters. It is an array (3, npts) where 0 is the x component , 1 is y component and 2 is the z components
- north, the unit vector in geocentric coordinates at the location in the direction of north. It is an array of (3, npts) and is same as location except it is a unit vector
- east, the unit vector in geocentric coordinates at the location in the direction of east. It is an array of (3, npts) and is same as location except it is a unit vector
- down, the unit vector in geocentric coordinates at the location in the direction of down. It is an array of (3, npts) and is same as location except it is a unit vector
Author: Daniel Letros Date Revised: 2017-02-24
-
classmethod