GEM-MACH¶
The GEM-MACH package provides a sasktran.Climatology and a ISKClimatology interface to the GEM-MACH 2.5 km regional climate model. It reads model output variables from netcdf4 generated by Chris McLinden’s group at Environment Canada. Note that the model has relatively coarse height resolution in the stratosphere
Quick Start¶
Here is an example that shows you how to use GEM-MACH. With a bit of luck this will be all you really need. There are two things specific to this climatology that must be done before you can use it to get height profiles,
You must tell the climatology which file to read with a call to set_gem_filename.
You must tell the climatology which species you are interested in so it will load the appropriate variables from file.
If you needmore info then you will have to read the rest of the documentation:
from datetime import datetime
import numpy as np
import matplotlib.pyplot as plt
from sasktran_gcm import GEM_MACH
climate = GEM_MACH() # Create the GEM-MACH object
climate.set_gem_filename(r'\\datastore\valhalla\root\data_products\GEM\20180621_gemmach2p5.nc') # Tell GEM-MACH which file to read.
climate.add_species ( 'SKCLIMATOLOGY_NO2_CM3') # Tell GEM-MACH which variables you want to load. You must do this before calling UpdateCache.
climate.add_species ( 'SKCLIMATOLOGY_NO2_VMR') # Add all the variables you want. Each variable added will require several seconds of processing in UpdateCache
latitude=50.3456289 # Set up a reference location
longitude=247.982739087 # The latitude, longitude and utc
utc=datetime.fromisoformat('2018-06-21T12:00:00.000000') # should be "inside" the GEM-MACH regional output ranges.
climate.update_cache(latitude,longitude, utc) # [Optional] Load up the cache. The time variable is used to load in two snapshots that straddle the location mjd
# All variables are now fixed. Only variables specified in `addvariable` are available
h = np.arange( 0, 60000.0, 100.0) # Prepare to extract profiles. Create a height resolution set of heights, 0 to 60 km
no2cm3 = climate.get_parameter( 'SKCLIMATOLOGY_NO2_CM3', latitude, longitude, h, utc) # Get a height profile of NO2 number density at the given location
no2vmr = climate.get_parameter( 'SKCLIMATOLOGY_NO2_VMR', latitude, longitude, h, utc) # Get a height profile of NO2 volume mixing ratio at the given location
plt.figure(1) # and plot the data plot the data.
plt.plot( no2vmr,h, 'ko-')
plt.figure(2)
plt.plot( no2cm3,h, 'ko-')
plt.show()
print('Done')
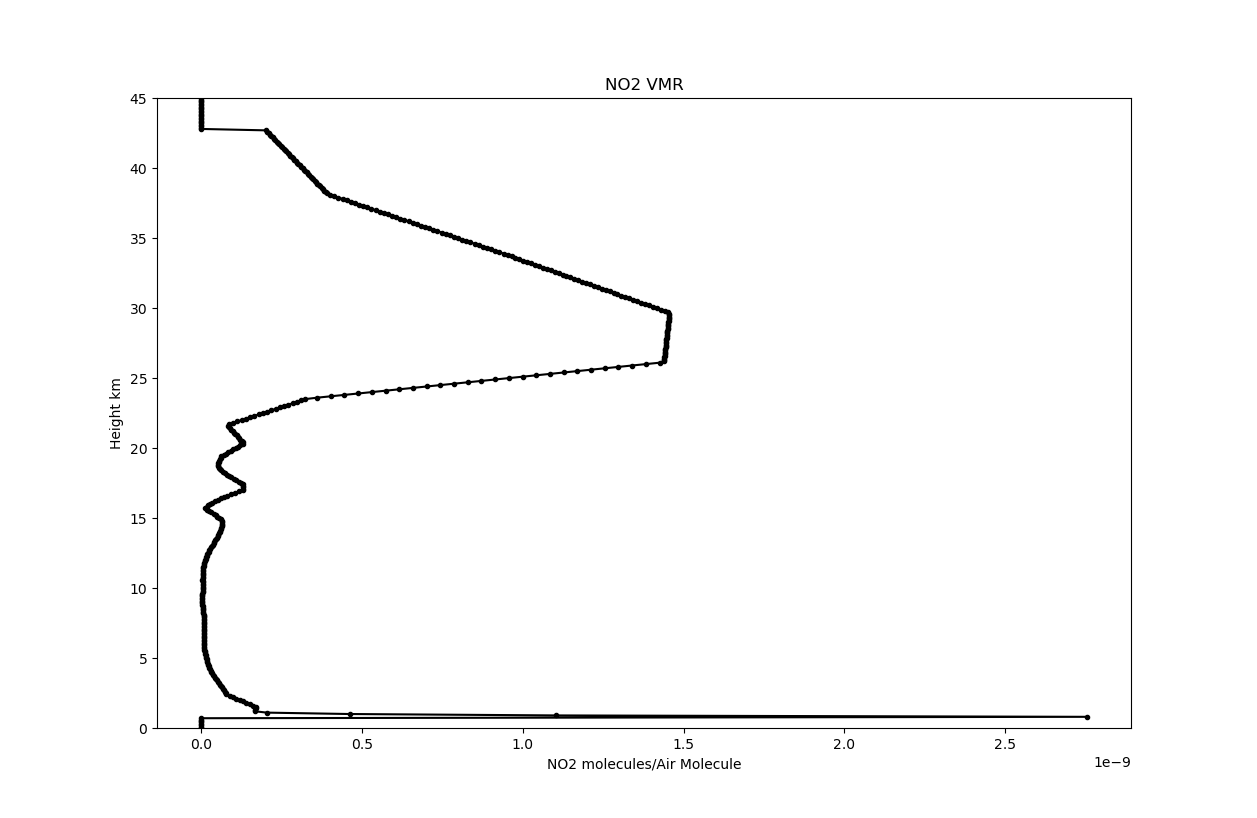
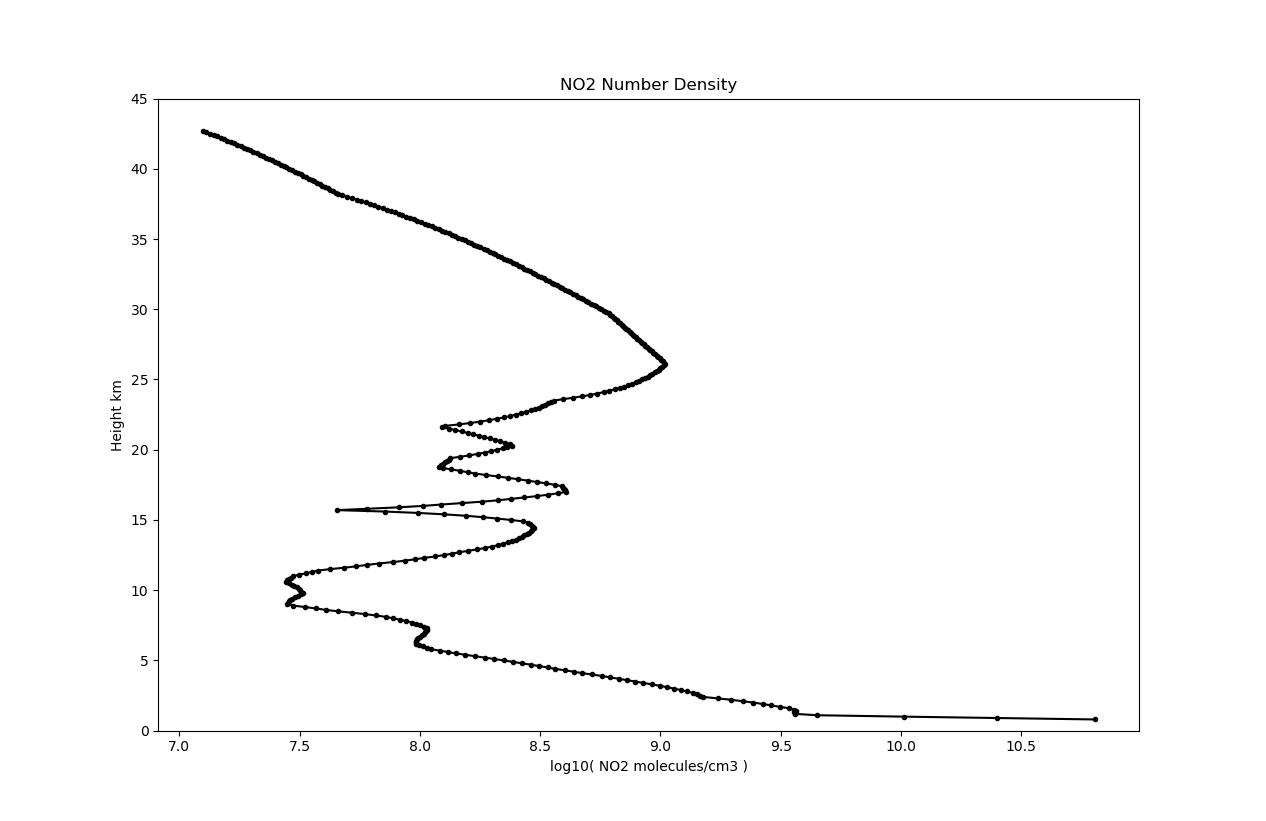
Sasktran Usage¶
The Sasktran GEM-MACH climatology is developed in C++ and complies with the ISKClimatology interface within the SasktranIF framework. A wrapper class has also been developed for use with the Sasktran API: import GEM_MACH from the sasktran_gcm package and create a new instance of class GEM_MACH:
>>> from sasktran_gcm import GEM_MACH
>>> gem = GEM_MACH()
>>> print(gem)
SasktranIF Climatology: GEM-MACH
Supported Species: ['SKCLIMATOLOGY_NO2_CM3', 'SKCLIMATOLOGY_AIRNUMBERDENSITY_CM3', 'SKCLIMATOLOGY_TEMPERATURE_K',
'SKCLIMATOLOGY_SURFACE_GEOMETRIC_HEIGHT', 'SKCLIMATOLOGY_SURFACE_GEOPOTENTIAL_HEIGHT',
'SKCLIMATOLOGY_CO_CM3', 'SKCLIMATOLOGY_CH4_CM3', 'SKCLIMATOLOGY_NH3_CM3', 'SKCLIMATOLOGY_NO_CM3',
'SKCLIMATOLOGY_O3_CM3', 'SKCLIMATOLOGY_SO2_CM3', 'SKCLIMATOLOGY_PRESSURE_PA',
'SKCLIMATOLOGY_NO2_VMR', 'SKCLIMATOLOGY_CO_VMR', 'SKCLIMATOLOGY_CH4_VMR', 'SKCLIMATOLOGY_NH3_VMR',
'SKCLIMATOLOGY_NO_VMR', 'SKCLIMATOLOGY_O3_VMR', 'SKCLIMATOLOGY_SO2_VMR']
>>>
GEM_MACH Class¶
-
class
sasktran_gcm.gem_mach_climatology.
GEM_MACH
¶ A climatology for the GEM-MACH 2.5 km regional model. The code works with the netcdf4 files generated by Chris McLindens group at Environment Canada.
Examples
>>> from sasktran_gcm import GEM_MACH >>> gem = GEM_MACH() >>> print(gem) SasktranIF Climatology: GEM-MACH Supported Species: ['SKCLIMATOLOGY_PRESSURE_PA', 'SKCLIMATOLOGY_AIRNUMBERDENSITY_CM3', 'SKCLIMATOLOGY_TEMPERATURE_K', 'SKCLIMATOLOGY_O2_CM3']
-
add_species
(speciesname)¶ Instructs the GEM-MACH climatology to load the requested variable when it next loads the internal cache. The function may be called multiple times to request multiple variables be loaded as long as all the calls are made before the first call to update_cache or get_parameter.
- Parameters
speciesname (str) – The name of any species supported by GEM-MACH. Complete list of species can be found in method supportedspecies of in the GEM-MACH documentation.
- Returns
Returns True if successful.
- Return type
bool
-
get_parameter
(species: str, latitude: float, longitude: float, altitudes: numpy.ndarray, utc: float) → numpy.ndarray¶ Get a profile from the climatology. The code will always update the internal cache unless the user has previously explicitly called update_cache. NOte that you must make all the necessary calls to set_gem_filename and add_species before the first call to get_parameter. Requesting to load species after the first call to update_cache or get_parameter is not supported.
- Parameters
species (str) – Species identifier of the species to query. Corresponds to
sasktran.Species.species
. The species must have been requested by a previous call to load_specieslatitude (float) – Latitude in degrees.
longitude (float) – Longitude in degrees.
altitudes (np.ndarray) – Profile altitude grid in meters.
mjd (float) – A scalar UTC that can be converted to Modified julian date. It can be a datetime, datetime64 or (mjd) number
- Returns
Climatology values at the specified altitudes.
- Return type
np.ndarray
-
set_gem_filename
(filename: str) → bool¶ Sets the filename that will be used for the GEM-MACH climatology. We currently do not have any standard naming conventions for the GEM-MACH files so the user must provide the name of the file they wish to use. This function must be called before the first call to update_cache or get_parameter otherwise the climatology will throw an exception when it tries to load the internal cache.
- Parameters
filename (str) – The full filename of the GEM-MACH netcdf file to be used for the climatology.
- Returns
Returns True if successful.
- Return type
bool
-
supported_species
()¶ Get a list of species identifiers that are supported by this climatology.
- Returns
Species identifiers that are supported by this climatology. Identifiers correspond to
sasktran.Species.species
- Return type
list
-
update_cache
(latitude: float, longitude: float, utc: float) → bool¶ Updates the GEM-MACH internal cache. Once this function is successfully called the cache will stay frozen in memory until the next call to update_cache. The GEM-MACH cache is currently updated if the UTC goes out of range of the current cache.
The user must make all the necessary calls to set_gem_filename and add_species before calling update_cache as only the species tagged for loading will be loaded into the cache.
- Parameters
latitude (float) – Latitude in degrees.
longitude (float) – Longitude in degrees.
mjd (float) – A scalar UTC that can be converted to Modified julian date. It can be a datetime, datetime64 or (mjd) number
- Returns
True if cache is successfully loaded.
- Return type
bool
-
SasktranIF Property List¶
Users may wish to program the gem mach climatology object directly through the SasktranIF interface:
ok = gem.skif_object.SetProperty('PropertyName', value)
The property name is not case sensitive, and the value may be a scalar, list, or array depending upon context
-
set_gem_filename
(string name)
¶ Specifies the filename of the GEM-MACH model that will be loaded at the next call to update_cache. Users must specify the full filename of the GEM-MACH file although it can be relative or absolute with respect to the current folder.
-
add_species
(string variablename)
¶ Specifies the name of a species to load at the next call to UpdateCache. Multiple variables can be requested using multiple calls to add_species but all calls must be made before the first call to update_cache or get_parameter.
Variables¶
The GEM-MACH package supports the following geophysical parameters,
Sasktran Handle
Description
SKCLIMATOLOGY_AIRNUMBERDENSITY_CM3
Air number density
SKCLIMATOLOGY_PRESSURE_PA
Pressure
SKCLIMATOLOGY_TEMPERATURE_K
Temperature
SKCLIMATOLOGY_SURFACE_GEOPOTENTIAL_HEIGHT
Surface Geopotential Height (meters)
SKCLIMATOLOGY_SURFACE_GEOMETRIC_HEIGHT
Surface Geometric Height (meters)
SKCLIMATOLOGY_CH4_CM3
CH4. Methane
SKCLIMATOLOGY_CH4_VMR
CH4. Methane
SKCLIMATOLOGY_CO_CM3
CO
SKCLIMATOLOGY_CO_VMR
CO
SKCLIMATOLOGY_NH3_CM3
NH3. Ammonia
SKCLIMATOLOGY_NH3_VMR
NH3. Ammonia
SKCLIMATOLOGY_NO2_CM3
NO2
SKCLIMATOLOGY_NO2_VMR
NO2
SKCLIMATOLOGY_NO_CM3
NO
SKCLIMATOLOGY_NO_VMR
NO
SKCLIMATOLOGY_O3_CM3
O3. Ozone
SKCLIMATOLOGY_O3_VMR
O3. Ozone
SKCLIMATOLOGY_O2_CM3
O2. Oxygen
SKCLIMATOLOGY_O2_O2_CM6
(O2)2for CIA
SKCLIMATOLOGY_SO2_CM3
SO2
SKCLIMATOLOGY_SO2_VMR
SO2
Variables are specified on 62 sigma pressure levels within the model across the rectangular region of interest. The system typically provides profiles that extend from the topographic surface to 40-45 km. The profiles have good height resolution at lower altitudes within the troposphere but become significantly coarser within the stratosphere. The current implementation provides no diagnostic information about the model height resolution to the user.
The variables in any given file are specified for one day at 24 hourly snapshots across a limited rectangular latitude-longitude area, typically 13 degrees by 12 degrees. The GEM-MACH sastran package implicitly assumes the user will limit their usage of the GEM-MACH model to the limited time period and regional area covered by the model. The current implementation provides no diagnostic information to the user about the temporal and spatial extents of the regional model. Users can use other tools, eg. xarray, Panoply or HDFView to obtain information not provided by the Sasktran GEM-MACH package.
Overview¶
Each GEM-MACH 2.5 km file provides a sequence of high spatial resolution snapshots of model output variables across a limited regional area. The area is typically of the order of ~13 degrees longitude by ~12 degrees latitude with 2.5 km resolution on a 62 level sigma level pressure grid. Snapshots are typically provided on the UTC hour for every hour in a specific day. For example, in the figure below we can see the geopotential height of the surface (in decimeters) across the regional area contained in an example GEM-MACH 2.5 km file.
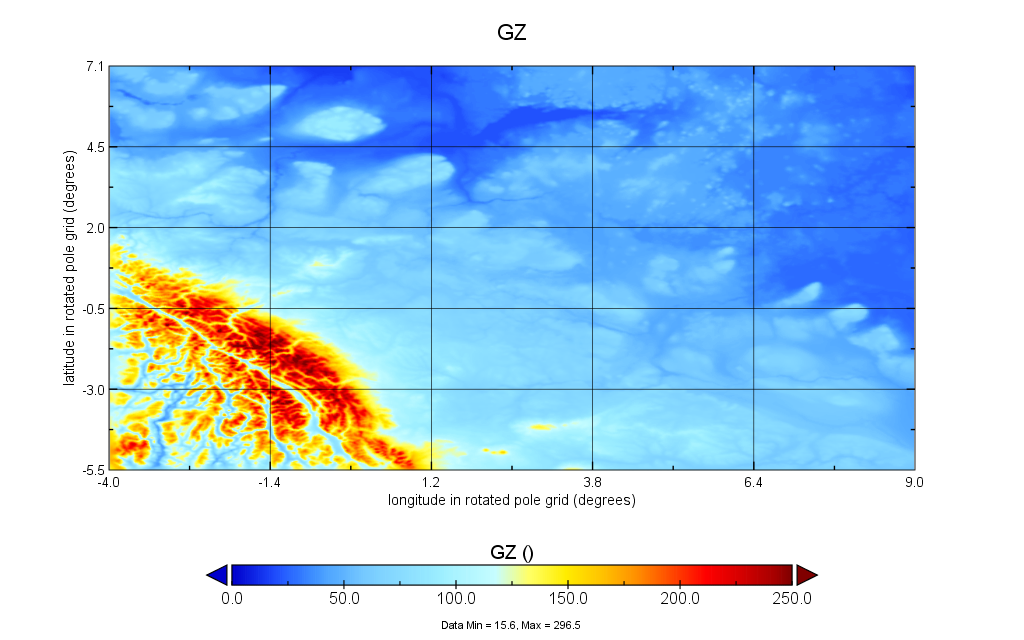
The grid covers approximately 13 degrees of longitude by 12.6 degrees of latitude. The internal coordinate system used by the GEM-MACH files is rotated_pole and once the regional area is mapped onto a geographic coordinate system we can from the image below see that the regional area is the lower half of Alberta Canada. The Rocky mountains are visible in the left hand corner of the regional plot above.
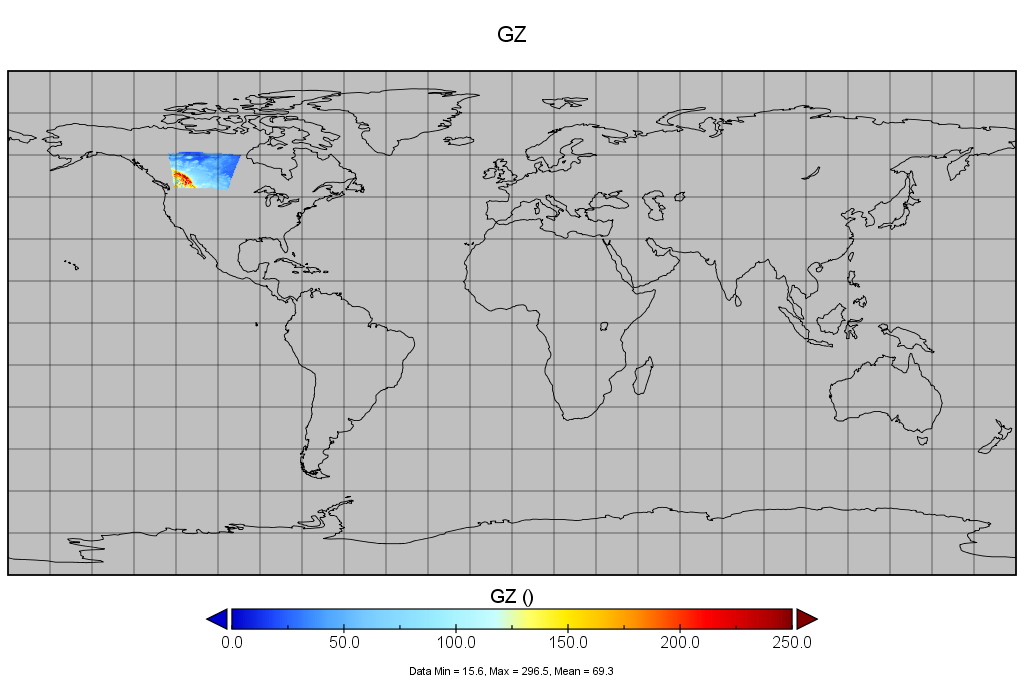
Time Extrapolation¶
The time range of any GEM-MACH variable is defined when calling UpdateCache. At this point two snapshots are loaded from the model output that straddle the requested time (UTC expressed as mjd). It is an error to request a time that is outside the time range spanned by the model.
Note
The GEM-MACH netcdf files only have 24 one hour samples which means it does not cover a full day. The files only cover the time from 00:00 UTC to 23:00 UTC on any specific day.
Time Interpolation¶
Any instant within the GEM-MACH time range is surround by two, hourly snapshots. Variables are calculated for each snapshot and then linearly interpolated in time. Linear interpolation occurs at a fixed geographic latitude, longitude and geometric height.
Horizontal Extrapolation¶
The GEM-MACH sasktran package allows users to ask for the value of variables outside the rectangular regional area but it will truncate results to the value at the appropriate border of the regional area. The intent of this rule is to proivide sensible support for the various secondary rays within a sasktran calculation that may stray beyond the limits of the GEM-MACH regional area.
Note
The user is responsible for ensuring they do not run significant amounts of their calculations outside the regional area of the GEM-MACH model. Such behaviour will not generate any warnings and may give “reasonable” but wrong results.
Horizontal Interpolation¶
All variables within the GEM-MACH regional area are linearly interpolated in the horizontal direction. Any geographic point on any hourly snapshot within the regional area is surrounded by the 4 points, two in latitude and two in longitude, and linearly interpolate din both directions. The horizontal interpolation is performed at a fixed geometric height.
Vertical Extrapolation¶
All variables within the sasktran GEM-MACH package are set to zero at all altitudes above the highest level of the model. All variables, except temperature, are set to zero for all altitudes below the topographic surface. Note that the topographic surface is the height of actual ground above sea-level and can change within the GEM-MACH regional area from 1.4 km in the Rocky mountains to 200 meters on the Prairies. This latter, sub-surface, condition allows the topographic conditions inherent to the GEM-MACH model to be incorporated, at least to first order, within the Sasktran radiative transfer models by approximating the atmosphere below the topographic surface as a vacuum.
Note
The “vacuum below the surface” approximation is not available in other sasktran climatologies. Users should ensure they have the appropriate, “below surface” behaviour in all climatologies used in any sasktran calculation otherwise strange results may occur.
Vertical Interpolation¶
Vertical interpolation of GEM-MACH variables, is to first order, arranged so that volume mixing ratios are linearly interpolated with altitude while number densities are log interpolated. In practice the interpolation of the number density parameters is more complicated. Number density parameters are not directly available in the GEM-MACH output and generated from interpolation of the appropriate volume mixing ratio, pressure and temperature. Volume mixing ratio and temperature are linearly interpolated while pressure is log interpolated.